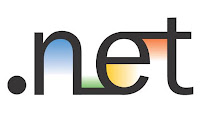
XElement represents an XML element. This post will describe about how to convert Dictionary to XML Format.
Assume you want the XML to be in the following format,
<paramlist><param><key>1</key><value>100</value></param><param><key>2</key><value>150</value></param></paramlist>
The Dictionary is having <String, String> Format with Two Entries inside as shown below.<key, 1>
<value, 100>
Now we have a List of dictionary as shown below,
List<Dictionary<string, string>>
Include the following Namespaces
using System.Collections.Generic;
using System.Xml.Linq;
using System.Xml.Linq;
Use the below method to convert the List<Dictionary> to XML (XElement)
Private XEement GetXML(List<Dictionary<string, string>> MyList)
{
XElement myXml = new XElement("paramList");
if (MyList != null)
{
foreach (Dictionary<string, string> entry in MyList)
{
myXml.Add(new XElement("param",
new XElement("key", entry["key"]),
new XElement("value", entry["value"])
));
}
}
if (MyList != null)
{
foreach (Dictionary<string, string> entry in MyList)
{
myXml.Add(new XElement("param",
new XElement("key", entry["key"]),
new XElement("value", entry["value"])
));
}
}
return myXml;
}
}
0 comments:
Post a Comment